Transfer Function Helper Tutorial¶
Here, we explain how to use TransferFunctionHelper to visualize and interpret yt volume rendering transfer functions. Creating a custom transfer function is a process that usually involves some trial-and-error. TransferFunctionHelper is a utility class designed to help you visualize the probability density functions of yt fields that you might want to volume render. This makes it easier to choose a nice transfer function that highlights interesting physical regimes.
First, we set up our namespace and define a convenience function to display volume renderings inline in the notebook. Using %matplotlib inline
makes it so matplotlib plots display inline in the notebook.
[1]:
import numpy as np
from IPython.core.display import Image
import yt
from yt.visualization.volume_rendering.transfer_function_helper import (
TransferFunctionHelper,
)
def showme(im):
# screen out NaNs
im[im != im] = 0.0
# Create an RGBA bitmap to display
imb = yt.write_bitmap(im, None)
return Image(imb)
Next, we load up a low resolution Enzo cosmological simulation.
[2]:
ds = yt.load("Enzo_64/DD0043/data0043")
Now that we have the dataset loaded, let’s create a TransferFunctionHelper
to visualize the dataset and transfer function we’d like to use.
[3]:
tfh = TransferFunctionHelper(ds)
TransferFunctionHelpler
will intelligently choose transfer function bounds based on the data values. Use the plot()
method to take a look at the transfer function.
[4]:
# Build a transfer function that is a multivariate gaussian in temperature
tfh = TransferFunctionHelper(ds)
tfh.set_field(("gas", "temperature"))
tfh.set_log(True)
tfh.set_bounds()
tfh.build_transfer_function()
tfh.tf.add_layers(5)
tfh.plot()
[4]:
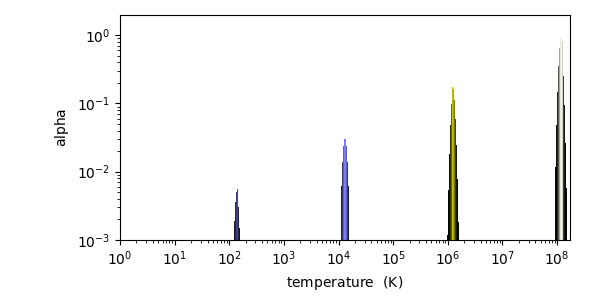
Let’s also look at the probability density function of the mass
field as a function of temperature
. This might give us an idea where there is a lot of structure.
[5]:
tfh.plot(profile_field=("gas", "mass"))
[5]:
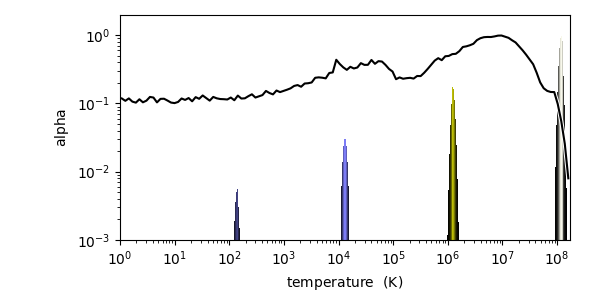
It looks like most of the gas is hot but there is still a lot of low-density cool gas. Let’s construct a transfer function that highlights both the rarefied hot gas and the dense cool gas simultaneously.
[6]:
tfh = TransferFunctionHelper(ds)
tfh.set_field(("gas", "temperature"))
tfh.set_bounds()
tfh.set_log(True)
tfh.build_transfer_function()
tfh.tf.add_layers(
8,
w=0.01,
mi=4.0,
ma=8.0,
col_bounds=[4.0, 8.0],
alpha=np.logspace(-1, 2, 7),
colormap="RdBu_r",
)
tfh.tf.map_to_colormap(6.0, 8.0, colormap="Reds")
tfh.tf.map_to_colormap(-1.0, 6.0, colormap="Blues_r")
tfh.plot(profile_field=("gas", "mass"))
---------------------------------------------------------------------------
ValueError Traceback (most recent call last)
Cell In[6], line 6
4 tfh.set_log(True)
5 tfh.build_transfer_function()
----> 6 tfh.tf.add_layers(
7 8,
8 w=0.01,
9 mi=4.0,
10 ma=8.0,
11 col_bounds=[4.0, 8.0],
12 alpha=np.logspace(-1, 2, 7),
13 colormap="RdBu_r",
14 )
15 tfh.tf.map_to_colormap(6.0, 8.0, colormap="Reds")
16 tfh.tf.map_to_colormap(-1.0, 6.0, colormap="Blues_r")
File /tmp/yt/yt/visualization/volume_rendering/transfer_functions.py:893, in ColorTransferFunction.add_layers(self, N, w, mi, ma, alpha, colormap, col_bounds)
891 elif alpha is None and not self.grey_opacity:
892 alpha = np.logspace(-3, 0, N)
--> 893 for v, a in zip(np.mgrid[mi : ma : N * 1j], alpha, strict=True):
894 self.sample_colormap(v, w, a, colormap=colormap, col_bounds=col_bounds)
ValueError: zip() argument 2 is shorter than argument 1
Let’s take a look at the volume rendering. First use the helper function to create a default rendering, then we override this with the transfer function we just created.
[7]:
im, sc = yt.volume_render(ds, [("gas", "temperature")])
source = sc.get_source()
source.set_transfer_function(tfh.tf)
im2 = sc.render()
showme(im2[:, :, :3])
[7]:
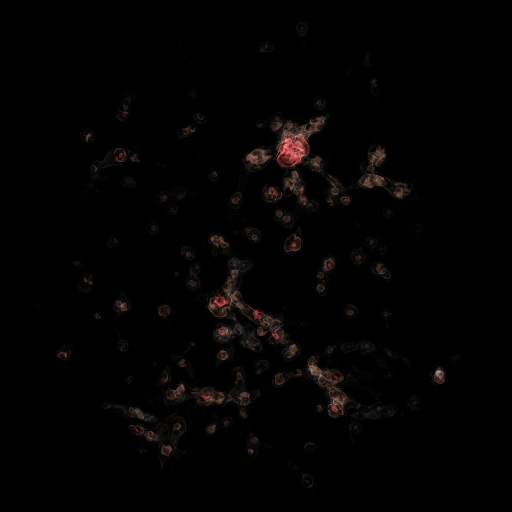
That looks okay, but the red gas (associated with temperatures between 1e6 and 1e8 K) is a bit hard to see in the image. To fix this, we can make that gas contribute a larger alpha value to the image by using the scale
keyword argument in map_to_colormap
.
[8]:
tfh2 = TransferFunctionHelper(ds)
tfh2.set_field(("gas", "temperature"))
tfh2.set_bounds()
tfh2.set_log(True)
tfh2.build_transfer_function()
tfh2.tf.add_layers(
8,
w=0.01,
mi=4.0,
ma=8.0,
col_bounds=[4.0, 8.0],
alpha=np.logspace(-1, 2, 7),
colormap="RdBu_r",
)
tfh2.tf.map_to_colormap(6.0, 8.0, colormap="Reds", scale=5.0)
tfh2.tf.map_to_colormap(-1.0, 6.0, colormap="Blues_r", scale=1.0)
tfh2.plot(profile_field=("gas", "mass"))
---------------------------------------------------------------------------
ValueError Traceback (most recent call last)
Cell In[8], line 6
4 tfh2.set_log(True)
5 tfh2.build_transfer_function()
----> 6 tfh2.tf.add_layers(
7 8,
8 w=0.01,
9 mi=4.0,
10 ma=8.0,
11 col_bounds=[4.0, 8.0],
12 alpha=np.logspace(-1, 2, 7),
13 colormap="RdBu_r",
14 )
15 tfh2.tf.map_to_colormap(6.0, 8.0, colormap="Reds", scale=5.0)
16 tfh2.tf.map_to_colormap(-1.0, 6.0, colormap="Blues_r", scale=1.0)
File /tmp/yt/yt/visualization/volume_rendering/transfer_functions.py:893, in ColorTransferFunction.add_layers(self, N, w, mi, ma, alpha, colormap, col_bounds)
891 elif alpha is None and not self.grey_opacity:
892 alpha = np.logspace(-3, 0, N)
--> 893 for v, a in zip(np.mgrid[mi : ma : N * 1j], alpha, strict=True):
894 self.sample_colormap(v, w, a, colormap=colormap, col_bounds=col_bounds)
ValueError: zip() argument 2 is shorter than argument 1
Note that the height of the red portion of the transfer function has increased by a factor of 5.0. If we use this transfer function to make the final image:
[9]:
source.set_transfer_function(tfh2.tf)
im3 = sc.render()
showme(im3[:, :, :3])
[9]:
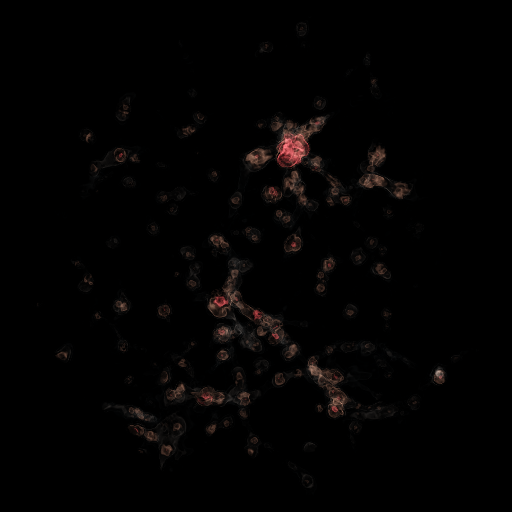
The red gas is now much more prominent in the image. We can clearly see that the hot gas is mostly associated with bound structures while the cool gas is associated with low-density voids.